메소드 오버라이딩
- 부모 클래스의 메소드를 자식 클래스에서 재정의하여 사용
- 부모 클래스의 메소드의 일부를 변경하여 사용하고 싶을 때 유용함
메소드 오버라이딩의 이해와 예제
- 앞서 상속에서 설명한 과일을 예제로 설명해 보겠다.
- 과일 클래스로 부터 상속받아 사과클래스, 바나나클래스 등을 정의했다.
- 과일 클래스의 메소드로 과일을 먹는 방법에 대하여 설명하는 메소드가 있다고 가정한다.
- 과일들은 대부분 씻어먹는 과일이 많기 때문에 '잘 씻어서 먹어야 합니다.'라고 출력하는 메소드를 부모클래스에서 정의했다.
- 하지만 바나나의 경우 씻어먹는 것이 아닌 껍질을 벗겨서 먹는 과일이다! 이럴 경우 메소드를 따로 하나 더 만드는 것도 방법이 될 수 있겠지만 기존 부모클래스의 메소드를 재정의 함으로써 코드의 통일감을 줄 수 있다.
앞서 상속에서 정의한 과일 클래스와 수입 클래스이다.
사과 클래스와 바나나클래스는 과일 클래스를 상속받고 있으며, 바나나 클래스는 수입 클래스도 상속받고 있다.
사과 클래스를 만들고 상속받은 메소드인 howtoeat을 호출해 보았다.
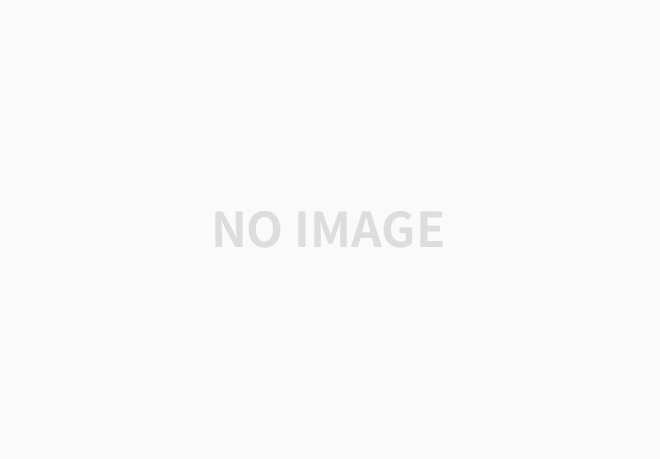
부모클래스로 부터 상속받은 howtoeat 메소드가 잘 호출되어 "잘 씻어서 먹어야 합니다."라는 문구가 출력된다.
하지만 바나나의 경우에는 씻어 먹는 것이 아닌 껍질을 벗겨 먹어야 한다. 이럴 경우 바나나 클래스에 메소드 오버라이딩을 해준다.
위와 같이 바나나 클래스에 부모클래스와 같은 이름인 howtoeat을 재정의 해주고 바나나에 맞는 문구를 작성해 준다.
바나나 객체를 만든 이후에 howtoeat() 메소드를 호출하니
부모클래스인 과일 클래스의 메소드가 아닌 자식클래스인 바나나 클래스의 메소드가 호출되는 모습을 볼 수 있다.
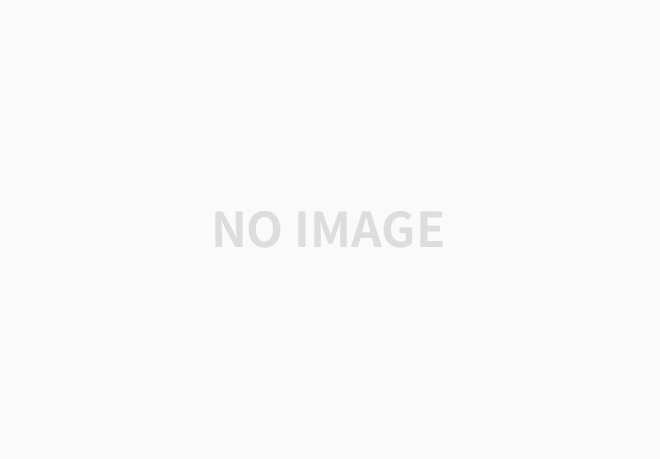
'IT > 파이썬 기초' 카테고리의 다른 글
[파이썬 기초] 상속, 다중상속 (5) | 2023.07.13 |
---|---|
[파이썬 기초] class, 생성자, 멤버변수, 메소드 정리 (0) | 2023.07.08 |
[파이썬 기초] with 문을 이용한 간단한 파일 입출력 (0) | 2023.07.07 |
[파이썬 기초] pickle 이용한 파일 입출력, 데이터 로딩 (0) | 2023.07.06 |
[파이썬 기초] 파일 입출력 (0) | 2023.07.05 |